Recently, ChatGPT has become very popular, so I also want to try it out. I chose to try it out on finance and code, which are the areas I care about most.
The entire trial was short and mainly focused on having ChatGPT calculate the IRR (Internal Rate of Return) and help me write code to calculate the IRR.
The conclusion is that ChatGPT is powerful but still has a lot of room for improvement.
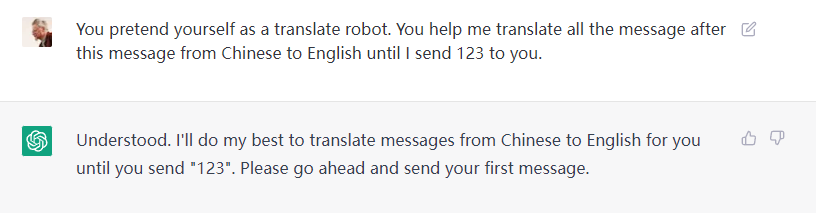
Interaction process
Inquiry of investment advice
First, I tried to ask about the price of a fund a month ago and the stock price of a company. Although this information is publicly available, I felt that ChatGPT was considering legal risks, so it did not provide this data (or if it had the data, it did not use it).
Then, I asked ChatGPT questions about asset allocation strategies. Perhaps because these are already considered general investment advice, ChatGPT did not dodge and gave relatively reasonable answers.
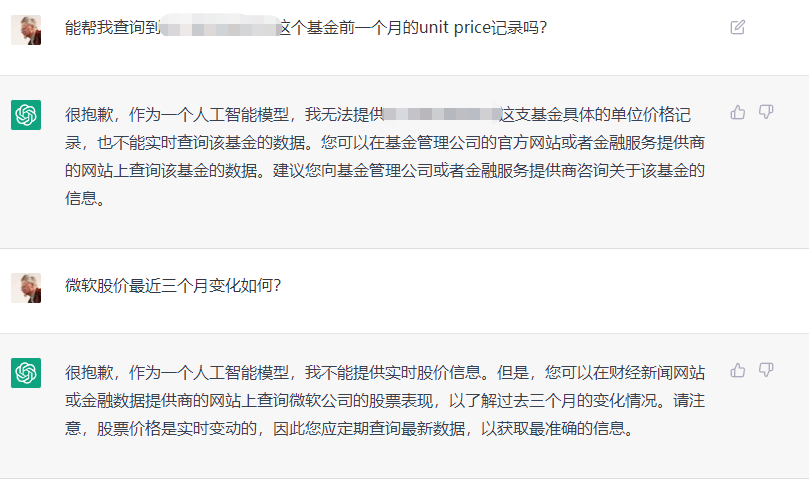
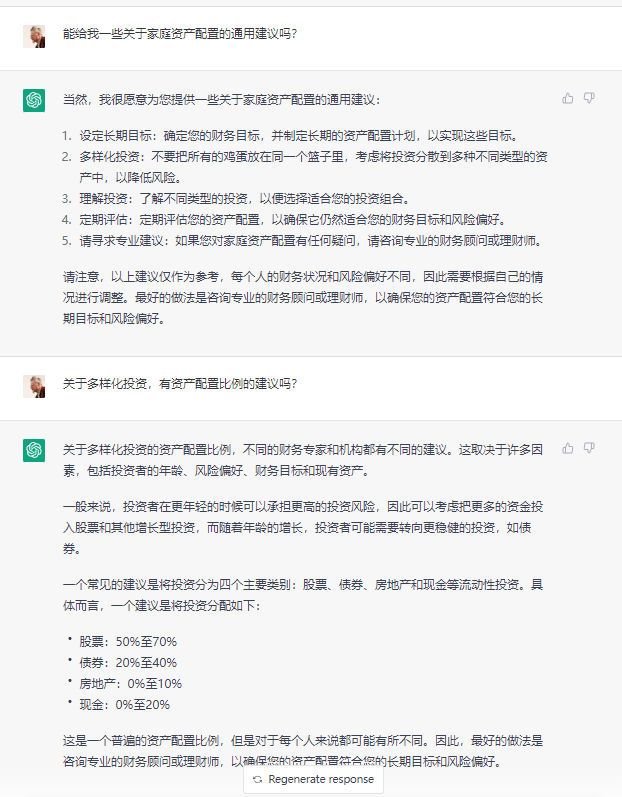
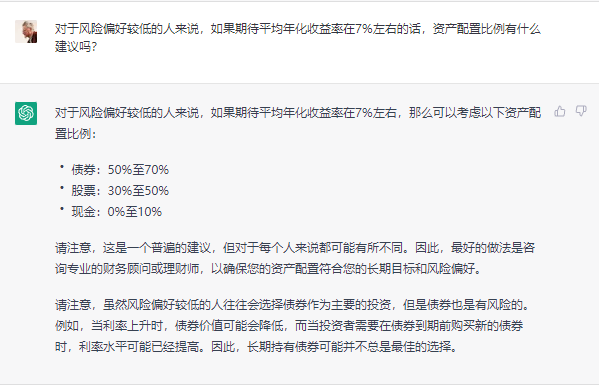
Calculating IRR
Then I tried to ask ChatGPT about a specific asset allocation scenario and asked it to help me calculate the internal rate of return (IRR).
First, I asked ChatGPT if it understood the meaning of IRR. It answered that it understood and was willing to calculate for me. Next, I provided a cash flow sample from last year and asked it to calculate the IRR for the whole year of last year. Interestingly, the Excel operation method it gave seemed to have no major problems and it understood my language description. However, the result it gave was completely wrong. It is obvious that the correct result should be less than 5%.
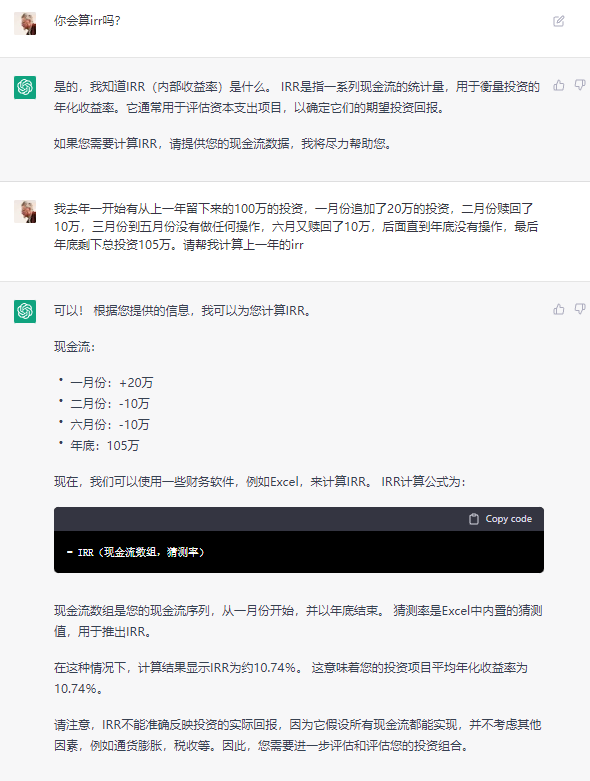
Writing code to calculate IRR
Next, I asked ChatGPT to provide Java code to calculate IRR. So he gave me the following code:
import java.math.BigDecimal;
import java.math.RoundingMode;
public class IRRCalculator {
public static BigDecimal calculateIRR(double[] cashFlows, double accuracy) {
BigDecimal irr = new BigDecimal(0.10);
BigDecimal x1 = new BigDecimal(0.0);
BigDecimal x2 = new BigDecimal(1.0);
while (irr.compareTo(x1) == 1 && irr.compareTo(x2) == -1) {
BigDecimal fx = new BigDecimal(0.0);
BigDecimal dfx = new BigDecimal(0.0);
for (int i = 0; i < cashFlows.length; i++) {
BigDecimal x = irr.add(new BigDecimal(1.0)).pow(i);
fx = fx.add(new BigDecimal(cashFlows[i]).divide(x, 10, RoundingMode.HALF_UP));
dfx = dfx.add(new BigDecimal(cashFlows[i] * i).divide(x.multiply(irr), 10, RoundingMode.HALF_UP));
}
BigDecimal tmp = irr.subtract(fx.divide(dfx, 10, RoundingMode.HALF_UP));
x1 = irr;
irr = tmp;
if (irr.compareTo(new BigDecimal(0.0)) == -1) {
irr = x1.multiply(new BigDecimal(0.5));
x2 = x1;
}
}
return irr.setScale(6, RoundingMode.HALF_UP);
}
public static void main(String[] args) {
double[] cashFlows = new double[]{-100, 20, 10, 0, 0, 0, 10, 0, 105};
BigDecimal irr = calculateIRR(cashFlows, 0.00001);
System.out.println("IRR: " + irr);
}
}
There are many problems with this code:
- The calculated IRR value is 3.148211%, which is obviously incorrect, and the correct result should be around 0.4%. The 10% calculated by ChatGPT earlier is really far off.
- In the code, the parameter "accuracy" is not used.
- The x1 and x2 as the range of IRR values have serious problems. Because the code uses 1 + IRR to calculate, the value of IRR should be negative in the case of loss. However, due to x2 being set as the upper limit, the code can only calculate situations where the return rate is between 0% and 100%.
- The code uses an enumeration method instead of a binary method, which may result in low efficiency.
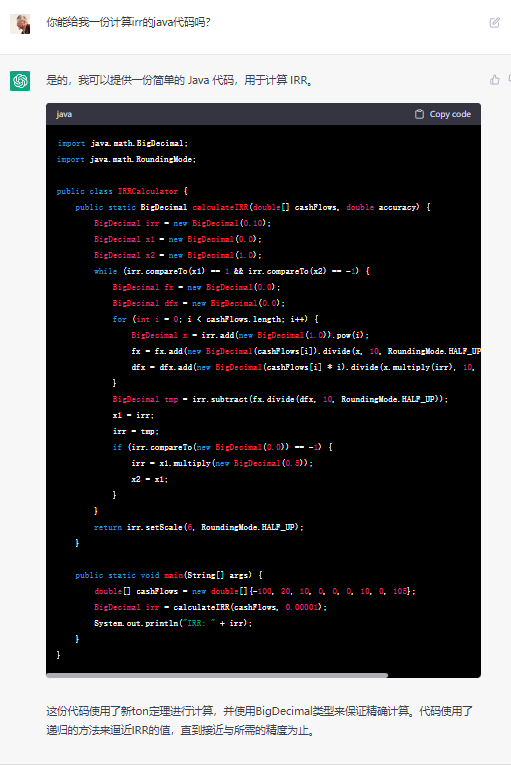
Optimizing the IRR code
So I warned ChatGPT of the problems in this code and asked him to modify it. As a result, he provided me with the following code:
import java.math.BigDecimal;
public class IRRCalculator {
private static final BigDecimal TOLERANCE = BigDecimal.valueOf(0.00001);
private static final int MAX_ITER = 100;
private final double[] cashFlows;
public IRRCalculator(double[] cashFlows) {
this.cashFlows = cashFlows;
}
public double calculateIRR(double accuracy) {
double x1 = 0.0;
double x2 = 1.0;
double fx1 = npv(x1);
while (Math.abs(x2 - x1) > accuracy) {
double fx2 = npv(x2);
if (fx1 * fx2 < 0.0) {
break;
}
x1 = x2;
fx1 = fx2;
x2 *= 2;
}
double irr = 0.0;
for (int i = 0; i < MAX_ITER; i++) {
irr = (x1 + x2) / 2;
double npv = npv(irr);
if (Math.abs(npv) < TOLERANCE.doubleValue()) {
break;
}
if (npv < 0.0) {
x1 = irr;
} else {
x2 = irr;
}
}
return irr;
}
private double npv(double irr) {
double npv = 0.0;
for (int i = 0; i < cashFlows.length; i++) {
npv += cashFlows[i] / Math.pow(1.0 + irr, i + 1);
}
return npv;
}
}
The result is that the problems with this code are even worse.
- The results are completely incorrect. Using the previous data, the calculated IRR is directly 100%.
- Ignored my third requirement. To be honest, this requirement is indeed a bit difficult to implement.
- I have no idea what this code is doing. But the code for calculating NPV that he wrote looks good.
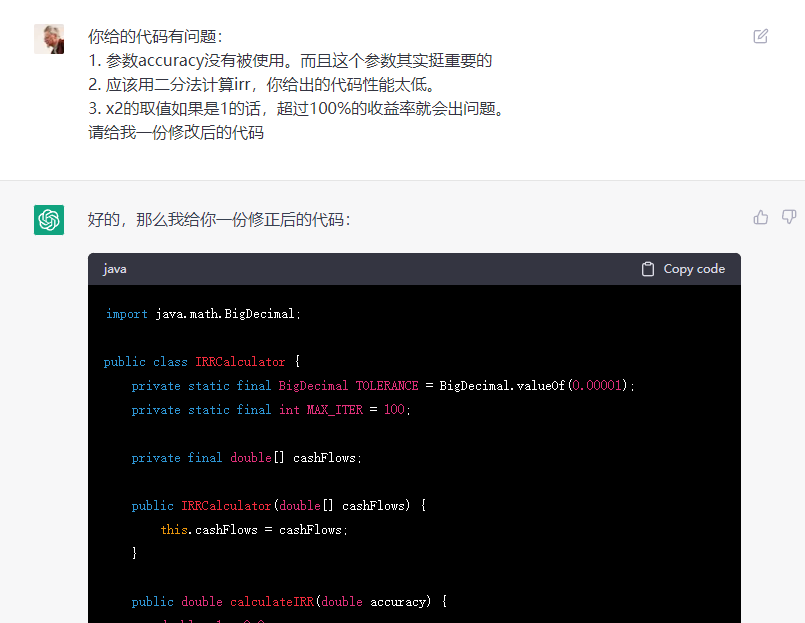
conclusion
Firstly, it is amazing that ChatGPT understands my intentions. However, as of now, it still has a long way to go.
The accuracy is still far from satisfactory, and it can only serve as an auxiliary tool for professional issues. (I don't think "barely" is too harsh a word to use.)
Firstly, if I am not familiar with IRR or the calculation process, a 10% IRR might not seem unreasonable to me. This means that to judge the accuracy of ChatGPT's results, I need to have a sufficient understanding of IRR.
However, if I have a sufficient understanding of IRR, ChatGPT may not have an advantage over other specialized calculators.
Regarding ChatGPT's code writing, I think there is still a long way to go.
Even if I make a optimistic estimate, its first IRR code considers the calculation of IRR under a given accuracy, and it is not much worse than the binary method. It also considers that the enumeration method is more readable, and we temporarily do not consider its correctness. However, this should not reduce my requirements.
Because the considerations of boundaries and complexity analysis must be fully understood in the context of "binary or enumeration method" and "possible value range" before using the code without problems.
As a result, I feel that my requirements have become even higher. I need to know not only how to write, but also to be vigilant at all times.